Python Programming Course – Learn to Code in Python
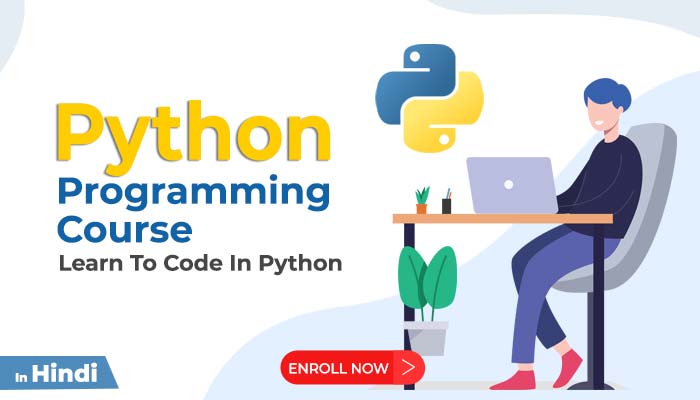
About Course
In our course on Python Programming Language, you get to learn many concepts related to Python, which is one of the most popular programming languages, and an easy-to-start-with language. We have made this course keeping the beginner students in mind. So, this course gets you smoothly from the beginning to many advanced concepts in an easy and handy way.
Throughout the course, we have tried to cover the concepts in an easy way, so that you can understand and implement the concepts. You earn a certificate for completion of the course, which has lifetime validity and can be shared on various employment platforms or on your CV and resume, or some other documents.
We always want the students to learn the updated things. So, we continuously keep updating the course, so that you can have the latest technology exposure.
At last, try to get the most out of the course. Try practicing because that is what many people do to become perfect. We wish you all the best and are excited to see you ahead in the course!
Course Content
Introduction to Python
-
Introduction to Python
01:13
Download Python
-
Download Python
03:20
Download Visual Studio Code
-
Download Visual Studio Code
07:08
Datatypes
-
Datatypes
06:38
Keywords
-
Keywords
05:44
Variables
-
Variables
11:05
comments
-
comments
08:45
Type Conversion
-
Type Conversion
13:57
Operators
-
introduction to operators
05:54 -
Arithmetic operators
12:13 -
Assignment operators
09:18 -
Comparison operators
11:44 -
logical operators
10:56 -
membership operators
04:40 -
operator precedence and associativity
17:23 -
Bitwise Operators
24:26 -
Identity Operators
14:17
Taking user input in python
-
Taking user input in python
11:43
Conditional statements (Decision making)
-
Introduction – if and else statements
12:26 -
Conditional statements program example – program for bigger number of two numbers
05:35 -
If elif statement
15:23 -
Nested if statements
04:25
Understanding Looping
-
Understanding for loop
17:48 -
Understanding while loop
08:47 -
break keyword
05:21 -
continue keyword
04:04 -
Using else with loops
05:43 -
Nested loops in Python
10:27
Assignment expression(Walrus operator)
-
Assignment expression(Walrus operator)
05:33
Understanding Strings
-
string introduction
12:44 -
String concatenation
03:56 -
Getting length of a string
04:35 -
accessing the string
10:28
String & String Methods
-
String Methods Introduction
02:01 -
String Methods – Upper Method
04:43 -
String Methods – Lower Method
03:36 -
String Methods – Capitalize Method
02:55 -
String Methods – Find Method
07:25 -
String Methods -Index Method
07:14 -
String Methods – Startswith Method
06:46 -
String Methods – Endswith Method
06:40
String Formatting
-
String Formatting
13:28
Structural Pattern Matching (Match – Case Statements)
-
Structural Pattern Matching (Match – Case Statements)
19:00
Understanding List
-
Introduction
05:52 -
getting the length of the list
02:34 -
Accessing the List
12:24 -
List can have data of Multiple Datatypes
02:38
List
-
List Methods Introduction
01:19 -
Append Method
03:08 -
Clear Method
02:44 -
copy method
04:37 -
Count Method
03:23 -
Index Method
04:35 -
Insert Method
03:11 -
Remove Method
04:34 -
Sort Method
04:34 -
Draft Lesson
05:49 -
Pop Method
05:49
List Comprehension in Python
-
List Comprehension in Python
11:30
Understanding tuple
-
How to Create a Tuple
06:41 -
Getting length of a tuple
02:53 -
Tuple with one Element
01:49
Tuple – Tuple Methods
-
Tuple methods introduction
01:35 -
Count Method
02:13 -
Index Method
03:47
Understanding dictionary
-
What is Dictionary
04:18 -
How to Create Dictionary
06:05 -
How to add Key Value Pairs to the Dictionary
09:39 -
Finding Length of Dictionary
02:51 -
how to delete some key value pair from the dictionary
04:50 -
creating complicated dictionaries
09:16 -
accessing the elements in the dictionary
10:34
Dictionary Methods
-
The clear method
03:03 -
The Copy Method
06:29 -
fromkeys method
09:15 -
Get Method
10:07 -
Items Method
05:05 -
keys method
04:57 -
pop method
04:42 -
popitem method
08:37 -
setdefault method
07:46 -
update method
08:45 -
values method
03:52
Dictionary – duplicate keys are not allowed in the dictionary
-
duplicate keys are not allowed in the dictionary
04:12
Dictionary – deleting the dictionary
-
deleting the dictionary
05:27
looping through dictionary
-
looping through dictionary
11:23
Functions
-
introduction to functions
16:22 -
advantages of using functions
09:48 -
what happens when we call the function
10:07 -
giving arguments to function
15:00 -
functions can return value
07:17 -
different argument types in function
11:02 -
default arguments
09:35 -
keyword arguments
06:03 -
variable number of arguments
10:42 -
variable number of keyword arguments
09:28 -
Lambda functions in python
11:04
Understanding set
-
introduction to set
08:07 -
calculating length of the set
02:01 -
accessing the elements
03:29
Set methods
-
set – methods introduction
01:48 -
add method
03:17 -
Clear Method
02:29 -
copy method
03:49 -
Remove Method
02:50 -
union method
04:33 -
intersection method
03:52 -
difference method
06:11
exception handling
-
Introduction to exception handling
08:45 -
Exploring exception and exception handling(try except block)
15:58 -
finally keyword
05:31 -
using else block with exception handling
04:33
file handling
-
introduction to file handling
02:13 -
creating a new file
06:28 -
how to write to some file
12:04 -
reading from a file
09:39 -
deleting a file
04:54
Math module
-
math module
01:00 -
math module constants
02:48 -
ceil function
03:41 -
floor function
03:45 -
factorial function
03:04 -
sqrt function
02:25 -
fabs function
02:52 -
pow function
02:35 -
exp2 function
02:23 -
log10 function
03:43 -
cbrt function
02:41
Object Oriented Programming
-
Introduction to Object Oriented Programming
05:06 -
What are classes and objects
16:28 -
What is constructor
11:12 -
Types of constructors
09:29
Object Oriented Programming – Inheritance
-
Inheritance explained
02:12 -
Implementing inheritance
10:34 -
single inheritance
03:05 -
multilevel inheritance
07:42 -
multiple inheritance
10:54
Object Oriented Programming – encapsulation
-
encapsulation
12:07
Object Oriented Programming – Polymorphism
-
introduction to polymorphism
01:36 -
method overloading in python
14:49 -
duck typing
13:11 -
operator overloading
10:28 -
method overriding
09:48
Object Oriented Programming – Access Modifiers
-
Access Modifiers
09:33
Object Oriented Programming – class methods
-
Class Methods
10:45
Object Oriented Programming – Static Methods
-
Static Methods
06:48
Outro – Youtube
-
Outro – Youtube
00:00
Collections Module
-
Collections Module Intro
01:47 -
Collections Counter
23:34 -
Collections Deque
22:12
Decorators in Python
-
Decorators in Python
33:19